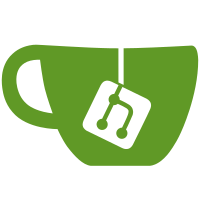
* Move code setting up the HTTP server to it's own package * This helps clean up the `main` function and make it easier to read * Rename the `BasicAuthMiddleware` to remove reference to Basic since it can now handle any type of auth type https://gitlab.com/hectorjsmith/fail2ban-prometheus-exporter/-/merge_requests/90
34 lines
772 B
Go
34 lines
772 B
Go
package server
|
|
|
|
import (
|
|
"log"
|
|
"net/http"
|
|
|
|
"github.com/prometheus/client_golang/prometheus/promhttp"
|
|
"gitlab.com/hectorjsmith/fail2ban-prometheus-exporter/collector/textfile"
|
|
)
|
|
|
|
const (
|
|
metricsPath = "/metrics"
|
|
)
|
|
|
|
func rootHtmlHandler(w http.ResponseWriter, r *http.Request) {
|
|
_, err := w.Write([]byte(
|
|
`<html>
|
|
<head><title>Fail2Ban Exporter</title></head>
|
|
<body>
|
|
<h1>Fail2Ban Exporter</h1>
|
|
<p><a href="` + metricsPath + `">Metrics</a></p>
|
|
</body>
|
|
</html>`))
|
|
if err != nil {
|
|
log.Printf("error handling root url: %v", err)
|
|
w.WriteHeader(http.StatusInternalServerError)
|
|
}
|
|
}
|
|
|
|
func metricHandler(w http.ResponseWriter, r *http.Request, collector *textfile.Collector) {
|
|
promhttp.Handler().ServeHTTP(w, r)
|
|
collector.WriteTextFileMetrics(w, r)
|
|
}
|